What is MySQL and Why is it so Important to Web Design?
Ronit Agarwal
. 1 min read
WordPress relies on the database management system known as MySQL to save and retrieve the information associated with your blog. It is a virtual filing cabinet that you can install on your website and its name is pronounced "my sequel." Even though you don't need to know how to use MySQL to use WordPress, having even a basic understanding of MySQL can be helpful when troubleshooting issues with your WordPress website. Here's an example of code that demonstrates a basic MySQL query within a WordPress context:
phpCopy code <?php// Establishing a MySQL database connection$servername = "localhost"; $username = "your_username"; $password = "your_password"; $database = "your_database"; $conn = new mysql($servername, $username, $password, $database); // Checking the connectionif ($conn->connect_error) { die("Connection failed: " . $conn->connect_error); } // Running a simple query to retrieve data from a WordPress table$sql = "SELECT * FROM wp_posts"; $result = $conn->query($sql); // Checking if the query returned any resultsif ($result->num_rows > 0) { // Outputting the datawhile ($row = $result->fetch_assoc()) { echo "Post ID: " . $row["ID"] . ", Title: " . $row["post_title"] . "<br>"; } } else { echo "No posts found."; } // Closing the database connection$conn->close(); ?>
In the above code, we establish a MySQL database connection using the provided credentials (replace 'your_username'
, 'your_password'
, and 'your_database'
with your actual database credentials). Then, we run a simple query to retrieve data from the WordPress table wp_posts
. The retrieved data is then looped through and outputted.
Note that this code is just an example and may need modifications based on your specific WordPress installation and requirements. It's important to handle database connections and queries securely and follow best practices when working with databases in WordPress.
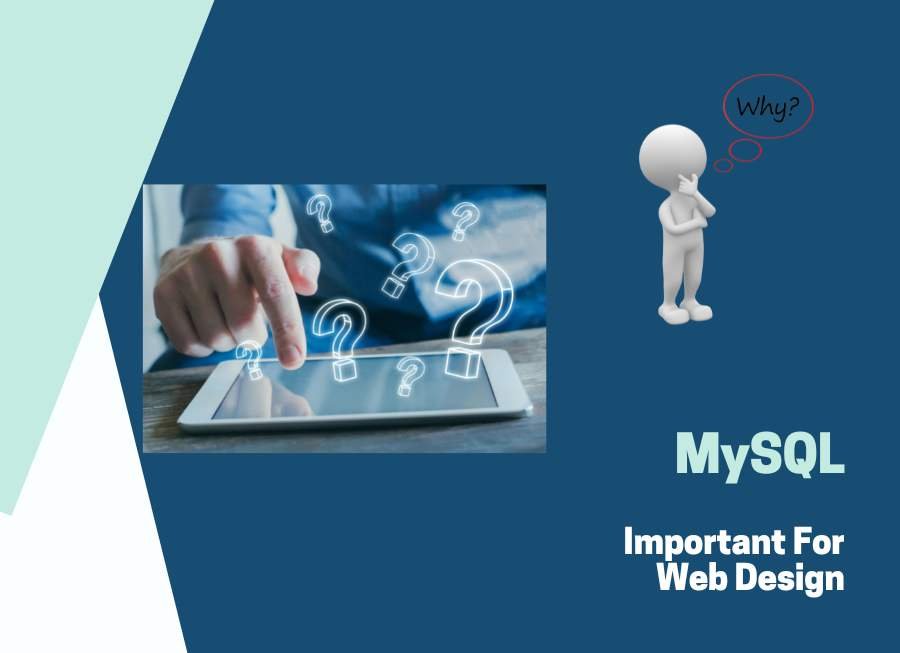
What exactly is a database?
MySQL is a relational database management system that can accommodate a large number of users and databases at once. It functions as a server and is installed on the hosting server that you use for WordPress. Imagine it as a virtual filing cabinet that helps you store, organize, and retrieve all of the information that is found on your website.
What Are the Different Ways That I Can Interact With the MySQL Database?
Simply being aware of what MySQL is not enough to win the battle. The other half is the one that is actually making use of it. The client-server architecture is what powers this database. This indicates that the user will engage in communication with the client in order to gain access to the server that is housing the data.
Advantages of Using MySQL for Programmers and Developers
The community of database and website developers has been very vocal about the solutions that they find to be most effective. MySQL is currently the second most popular database, trailing only Oracle in terms of user engagement as of June 2020.
MySQL has a wide range of compatibility
MySQL was developed to be highly compatible with a variety of other technologies and architectural styles, despite the fact that it is most commonly associated with web applications or internet services. The RDBMS is compatible with all of the major computing platforms, including Unix-based operating systems.
The Primary Advantages of Employing PHP and MySQL When Constructing Websites
PHP and MySQL are currently the open-source database management systems and scripting languages that have the most widespread use. As PHP is a server-side scripting language, it enables significant dynamic pages to be created, complete with individualized characteristics. One of the primary advantages of utilizing PHP and MySQL is that it makes it possible to create a website that is not only user-friendly and interactive.
More Stories from
English Grammar: A Comprehensive Guide
This article provides a comprehensive guide to English grammar, covering essential topics such as parts of speech, sentence structure, tenses, and common grammatical errors.
Improving Your Writing Skills: A Comprehensive Review of Grammarly Premium
This article discusses the benefits of using Grammarly, a grammar checker and writing tool, and whether or not the premium version is worth the investment.
Canva: Empowering Creativity and Design Simplicity
Discover how Canva, the user-friendly graphic design platform, has revolutionized the world of visual communication.
The Rise of Virtual Travel: Exploring the World from the Comfort of Home
This article explores the surging popularity of virtual travel, a modern phenomenon that allows individuals to experience the world's wonders from the comfort of their homes.
The Art of Photography: Capturing Moments that Last a Lifetime
In this article, we explore the fascinating world of photography, tracing its evolution from its early beginnings to the digital age.